Post
Share your knowledge.
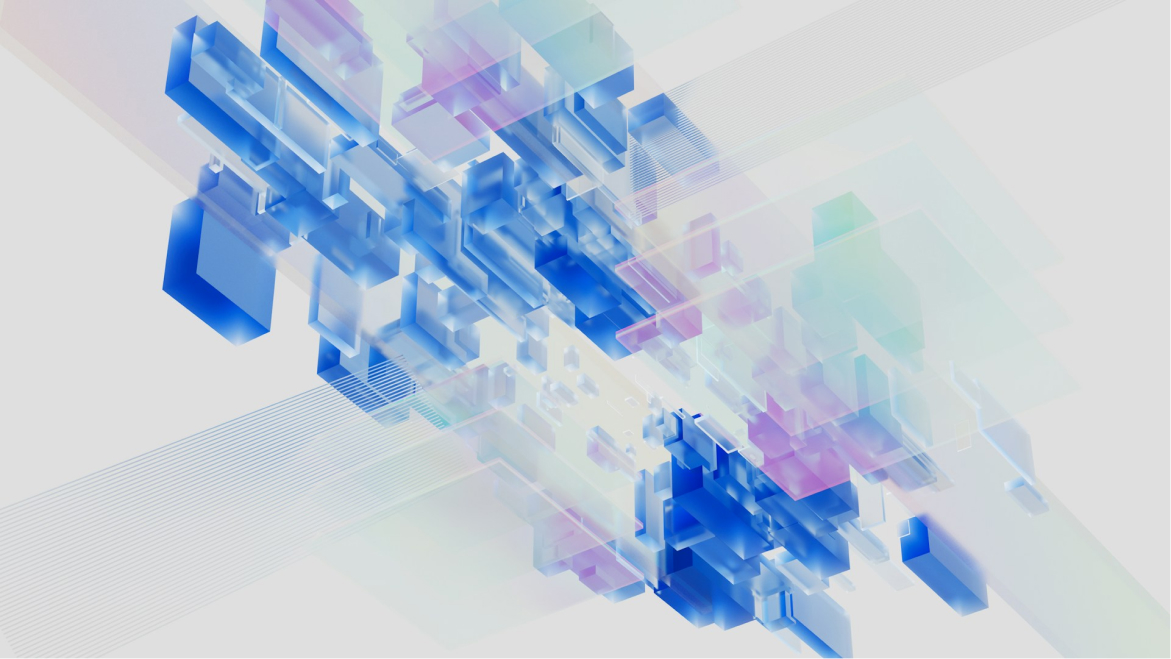
How to Build a Complex dApp on Sui Move?
Course #2: Deep Dive into Move Programming – Building Complex dApps on Sui
Now that you’ve grasped the basics of Move programming and deployed your first smart contract, it’s time to take your skills to the next level. In this article, we’ll explore how to build more complex decentralized applications (dApps) using Move on the Sui blockchain.
Step 1: Mastering Advanced Sui Move Concepts
Before diving into coding, let’s revisit some advanced features of Move that make it uniquely suited for building secure and scalable dApps:
1. Resource-Oriented Programming
Move treats digital assets as resources, ensuring they cannot be duplicated, unintentionally deleted, or misused (https://docs.sui.io/learn/resource-oriented-programming). This is achieved through strict ownership rules and type safety. For example:
module examples::token {
use sui::object::{Self, UID};
use sui::transfer;
struct Token has key, store {
id: UID,
value: u64,
}
public fun mint(ctx: &mut TxContext, value: u64): Token {
Token {
id: object::new(ctx),
value,
}
}
public fun transfer_token(token: Token, recipient: address) {
transfer::public_transfer(token, recipient);
}
}
In this example, the Token
resource is created and transferred securely. Resources in Move are immutable by default unless explicitly marked as mutable, adding an extra layer of security.
2. Modules and Encapsulation
Modules in Move act as self-contained units of functionality, enabling better organization and reusability. For instance, you can separate token creation logic from transfer logic into distinct modules (https://examples.sui.io/modules). This modularity ensures cleaner code and easier maintenance.
3. Object-Centric Design
Sui Move introduces an object-centric model, where every resource has a globally unique identifier (UID
). This allows for direct referencing and interaction with objects, making it easier to manage complex state transitions (https://docs.sui.io/objects).
Step 2: Writing a Modular Smart Contract
Let’s create a more advanced smart contract that demonstrates these concepts. We’ll build a simple NFT marketplace where users can mint and trade NFTs.
Define the NFT Resource
Start by defining an NFT resource within a Move module:
module examples::nft_marketplace {
use sui::object::{Self, UID};
use sui::transfer;
struct NFT has key, store {
id: UID,
name: String,
price: u64,
}
public fun mint_nft(ctx: &mut TxContext, name: String, price: u64): NFT {
NFT {
id: object::new(ctx),
name,
price,
}
}
public fun list_for_sale(nft: NFT, price: u64, ctx: &mut TxContext) {
nft.price = price;
transfer::public_transfer(nft, tx_context::sender(ctx));
}
}
Here, the NFT
resource includes properties like name
and price
. The mint_nft
function creates a new NFT, while list_for_sale
allows users to list their NFTs for sale.
Compile and Deploy
Use the Sui CLI to compile and deploy your contract. Write a deployment script to automate this process:
sui move build
sui client publish --gas-budget 10000
This will package and deploy your module to the Sui Devnet (https://docs.sui.io/cli).
Step 3: Building a React Frontend for Your Marketplace
With your smart contract deployed, let’s connect it to a React frontend.
Set Up the Project
Initialize a React project if you haven’t already:
npx create-react-app nft-marketplace
cd nft-marketplace
npm install @mysten/sui.js
Integrate with Sui Wallet
Use the @mysten/sui.js
library to interact with the Sui blockchain:
import { JsonRpcProvider, SuiClient } from '@mysten/sui.js';
const provider = new SuiClient({ url: 'https://fullnode.devnet.sui.io' });
async function fetchNFTs(ownerAddress) {
const objects = await provider.getObjectsOwnedByAddress(ownerAddress);
console.log('User NFTs:', objects);
}
Display NFT Data
Fetch and display NFT data in your React app:
function NFTList({ ownerAddress }) {
const [nfts, setNFTs] = useState([]);
useEffect(() => {
async function loadNFTs() {
const response = await provider.getObjectsOwnedByAddress(ownerAddress);
setNFTs(response.data);
}
loadNFTs();
}, [ownerAddress]);
return (
<div>
{nfts.map((nft) => (
<div key={nft.objectId}>
<p>{nft.name}</p>
<p>Price: {nft.price} SUI</p>
</div>
))}
</div>
);
}
Step 4: Enhancing Security and Performance
1. Secure Transactions
Ensure all transactions are validated both on-chain and off-chain. Use libraries like @mysten/sui.js
to verify transaction receipts:
async function verifyTransaction(txDigest) {
const result = await provider.getTransaction({ digest: txDigest });
console.log('Transaction Verified:', result);
}
2. Optimize Gas Fees
Partner with services like Shami Gas Station to offer gasless transactions, improving user experience. Alternatively, batch transactions to reduce costs (https://docs.sui.io/gas-optimization).
3. Leverage Sui’s Scalability
Sui’s architecture supports high throughput and low latency, making it ideal for dApps with heavy usage. Test your application under simulated load conditions to ensure performance remains consistent (https://performance.sui.io).
Step 5: Testing and Debugging
Testing is critical to avoid vulnerabilities. Use tools like Sui Explorer to monitor transactions and debug issues. Additionally, write unit tests for your Move modules:
#[test]
fun test_mint_nft() {
use sui::test_scenario;
let ctx = &mut test_scenario::ctx();
let nft = examples::nft_marketplace::mint_nft(ctx, "Test NFT", 100);
assert!(nft.price == 100, 0);
}
Run your tests using the Sui CLI:
sui move test
Step 6: Engaging with the Community
Building dApps is not just about coding—it’s also about collaboration. Share your progress on platforms like GitHub, Discord, or Twitter. Participate in hackathons and developer challenges hosted by the Sui Foundation to refine your skills and gain exposure.
Conclusion
By mastering advanced Move concepts, writing modular smart contracts, and building intuitive frontends, you’re well on your way to becoming a proficient dApp developer on the Sui blockchain. Remember to prioritize security, optimize performance, and engage with the community to maximize your impact.
Stay tuned for Course #3, where we’ll explore real-world use cases and advanced techniques for scaling your dApps on Sui!
If you’d like further clarification or additional resources, feel free to ask!
- Sui
- Architecture
- Move
Sui is a Layer 1 protocol blockchain designed as the first internet-scale programmable blockchain platform.
- Why does BCS require exact field order for deserialization when Move structs have named fields?53
- Multiple Source Verification Errors" in Sui Move Module Publications - Automated Error Resolution43
- Sui Transaction Failing: Objects Reserved for Another Transaction25
- How do ability constraints interact with dynamic fields in heterogeneous collections?05